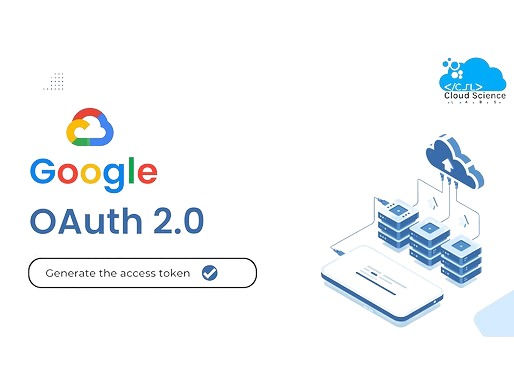
We will guide you to generate the access token with the help of Google OAuth 2.0 endpoint.
How does Google OAuth 2.0 endpoint work?
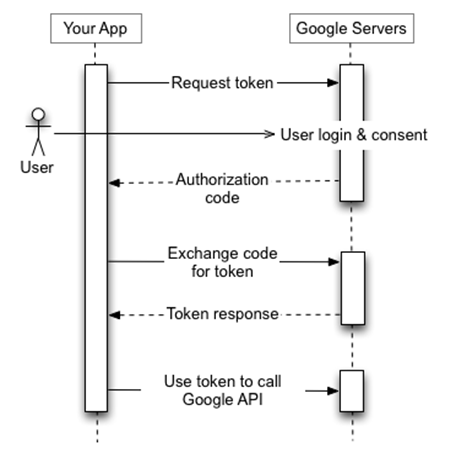
The Google OAuth 2.0 endpoint supports applications that are installed on devices such as computers, mobile devices, and tablets. When you create a client ID through the Google API Console, specify that this is an Installed application, then select Android, Chrome app, iOS, Universal Windows Platform (UWP), or Desktop app as the application type.
The process results in a client ID and, in some cases, a client secret, which you embed in the source code of your application.
The authorization sequence begins when your application redirects a browser to a Google URL; the URL includes query parameters that indicate the type of access being requested. Google handles the user authentication, session selection, and user consent. The result is an authorization code, which the application can exchange for an access token and a refresh token.
The application should store the refresh token for future use and use the access token to access a Google API. Once the access token expires, the application uses the refresh token to obtain a new one.
What query parameters do you need in the URL to send the Request?
Method: GET
Scope: scope=https://www.googleapis.com/auth/devstorage.read_write (Allows the access for only read and write).
Response Type: response_type=code
Access Type: access_type=offline
Client Id: Copy it from the Google Dev Console. (Please follow the above steps to find the client Id).
Redirect Url: Use System.Url.getOrgDomainUrl().toExternalForm() in your apex method to get the redirect URI
Complete URL:
Response:
{
"access_token": "ya29.a0AX9GBdUISkA7w3-VvES7CeK1vpYYAtdWSarqN7aahJAKsLbeRXDRNX1dmBnGAQcVNybIorUdx5rXolh18fhauPGOVlGWIWA7H7lovEO-YDTwmqxyjKdEAy3QG2FZEA_Zfv5_L9pVQGqIBFTg3-8Ut0An6ObwaCgYKASUSARASFQHUCsbCnxtJbObFWyO0CNN9zT5liQ0163",
"scope": "https://www.googleapis.com/auth/devstorage.read_write",
"token_type": "Bearer",
"expires_in": 3599,
"refresh_token": "1//04Tq09KPgq2MPCgYIARAAGAQSNwF-L9IrohqjYOdV1Gan8__nyW1b3XQEXAvoPH6Gp8nj3pQH_WVEJzVF4juhVxbqdOnkJ5CWKnE"
}
Note: Above URL has fake clientId and redirect URI and the response is also customized but response will have same keys with different values.
Hence, in this way you can get the access and refresh token.
How to upload an object to a GCP storage bucket? (File size must be smaller than 4MB
API URL:
Blob:
Blob file = EncodingUtil.base64Decode(fileBase64Content);
Note: fileBase64Content contains the base64 of a file or object.
Endpoint:
https://www.googleapis.com/upload/storage/v1/b/{bucketName}/o?uploadType=media&name={ORG_ID}/{Encodingutil.urlEncode(fileRecordId, 'UTF-8')}/{Encodingutil.urlEncode(filePath, 'UTF-8')}/{Encodingutil.urlEncode(Encodingutil.urlEncode(fileName, 'UTF-8'),'UTF-8')}
Note: We are encoding the filePath and fileName so that if it contains any special characters then it will easily encode them.
Access Token:
Above steps will guide you to how to get the Access token, follow them and get the access token.
Method: POST
REQUEST:
HttpRequest request = new HttpRequest();
request.setMethod(METHOD);
request.setEndpoint(Endpoint);
request.setHeader('Authorization','Bearer'+' '+ accessToken);
request.setHeader('Content-Type', 'multipart/mixed');
request.setHeader('Accept','*/*');
request.setHeader('Content-Type', ‘text/plain’);
request.setHeader('Content-length', String.valueof(file.size()));
request.setBodyAsBlob(file);
How to rename an object on a GCP storage bucket?
API URL:
Method: POST
File Path:
String existingPath = ORG_ID +'/'+ Encodingutil.urlEncode(recordId, 'UTF-8') +'/'+ Encodingutil.urlEncode(folderPath,'UTF-8') +'/'+ Encodingutil.urlEncode(Encodingutil.urlEncode(existingFile, 'UTF-8'),'UTF-8');
String newPath = ORG_ID +'/' + Encodingutil.urlEncode(recordId, 'UTF-8') +'/'+ Encodingutil.urlEncode(folderPath,'UTF-8') +'/'+ Encodingutil.urlEncode(Encodingutil.urlEncode(newFile, 'UTF-8'),'UTF-8');
Note: folderPath is the path of the file where it is stored. ExistingFile is the old name of that file which you requested to rename and newFile is the new name of that file.
Endpoint:
https://storage.googleapis.com/storage/v1/b/{bucketName}+ '/o/'+ existingPath.replace('/', '%2f')+ '/rewriteTo/b/'+ bucketName + '/o/'+ newPath.replace('/','%2f');
Access Token:
Above steps will guide you to how to get the Access token, follow them and get the access token.
Request:
HttpRequest request = new HttpRequest();
request.setMethod(METHOD);
request.setEndpoint(Endpoint);
request.setHeader('Authorization','Bearer'+' '+ accessToken);
request.setHeader('Content-Type', 'multipart/mixed');
request.setHeader('Accept','*/*');
request.setHeader('Content-Type', 'application/x-www-form-urlencoded');
request.setHeader('Content-Length', '0');
Note: Now we need to use the delete Api.
API URL:
Method: DELETE
File Path:
String path = ORG_ID +'/'+ Encodingutil.urlEncode(fileRecordId, 'UTF-8') +'/'+ Encodingutil.urlEncode(folderPath,'UTF-8') +'/'+ Encodingutil.urlEncode(Encodingutil.urlEncode(fileName, 'UTF-8'),'UTF-8');
Endpoint:
https://storage.googleapis.com/storage/v1/b/{bucketname}/o/{path.replace('/', '%2f')};
Request:
HttpRequest request = new HttpRequest();
request.setMethod(METHOD);
request.setEndpoint(Endpoint);
request.setHeader('Authorization','Bearer'+' '+ accessToken);
request.setHeader('Content-Type', 'multipart/mixed');
request.setHeader('Accept','*/*');
request.setHeader('Content-Type', 'application/x-www-form-urlencoded');
How to delete an object from GCP storage Bucket?
API URL:
Method: DELETE
File Path:
https://storage.googleapis.com/storage/v1/b/{bucketname}/o/{path.replace('/', '%2f')};
Request:
HttpRequest request = new HttpRequest();
request.setMethod(METHOD);
request.setEndpoint(Endpoint);
request.setHeader('Authorization','Bearer'+' '+ accessToken);
request.setHeader('Content-Type', 'multipart/mixed');
request.setHeader('Accept','*/*');
request.setHeader('Content-Type', 'application/x-www-form-urlencoded');
How to move an object from one location to another in a GCP storage bucket?
API URL:
Method: POST
File Path:
Note: moveFromFolder and moveToFolder are the folder paths.
String existingPath = ORG_ID +'/' + recordId +'/'+moveFromFolder + '/' + Encodingutil.urlEncode(fileName,'UTF-8');
String newPath = ORG_ID +'/' + recordId +'/'+moveToFolder + '/' + Encodingutil.urlEncode(fileName,'UTF-8');
Endpoint:
https://storage.googleapis.com/storage/v1/b/{bucketName} + '/o/'+{Encodingutil.urlEncode(existingPath, 'UTF-8')} + '/rewriteTo/b/'+ {bucketName} + '/o/'+ {Encodingutil.urlEncode(newPath, 'UTF-8')};
Request:
HttpRequest request = new HttpRequest();
request.setMethod(METHOD);
request.setEndpoint(Endpoint);
request.setHeader('Authorization','Bearer'+' '+ accessToken);
request.setHeader('Content-Type', 'multipart/mixed');
request.setHeader('Accept','*/*');
request.setHeader('Content-Type', 'application/x-www-form-urlencoded');
request.setHeader('Content-Type', 'application/x-www-form-urlencoded');
request.setHeader('Content-length', '0');
Note: Now we need to use the delete Api
Method: DELETE
File Path:
String path = ORG_ID +'/'+ Encodingutil.urlEncode(fileRecordId, 'UTF-8') +'/'+ Encodingutil.urlEncode(moveFromFolder,'UTF-8') +'/'+ Encodingutil.urlEncode(Encodingutil.urlEncode(fileName, 'UTF-8'),'UTF-8');
Endpoint:
https://storage.googleapis.com/storage/v1/b/{bucketname}/o/{path.replace('/', '%2f')};
Request:
HttpRequest request = new HttpRequest();
request.setMethod(METHOD);
request.setEndpoint(Endpoint);
request.setHeader('Authorization','Bearer'+' '+ accessToken);
request.setHeader('Content-Type', 'multipart/mixed');
request.setHeader('Accept','*/*');
request.setHeader('Content-Type', 'application/x-www-form-urlencoded');
How to preview an object or file which is present in our GCP Storage bucket?
When you upload a file or an object you get a medialink of that file or object in the response. You need to use that medialink as the endpoint when you send the request.
EndPoint:
MediaLink will be the endpoint.
Method: GET
Request:
HttpRequest request = new HttpRequest();
request.setEndpoint(Endpoint);
request.setMethod(METHOD);
request.setHeader('Authorization', 'Bearer'+' ' +accessToken);
How can you use the response to preview a file or object?
HttpResponse res = http.send(request);
Blob image = res.getBodyAsBlob();
String base64= EncodingUtil.base64Encode(image);
NOTE: Now use this base64 in your img src tag to preview your file. Also, you can use iframe for media files.
Comments